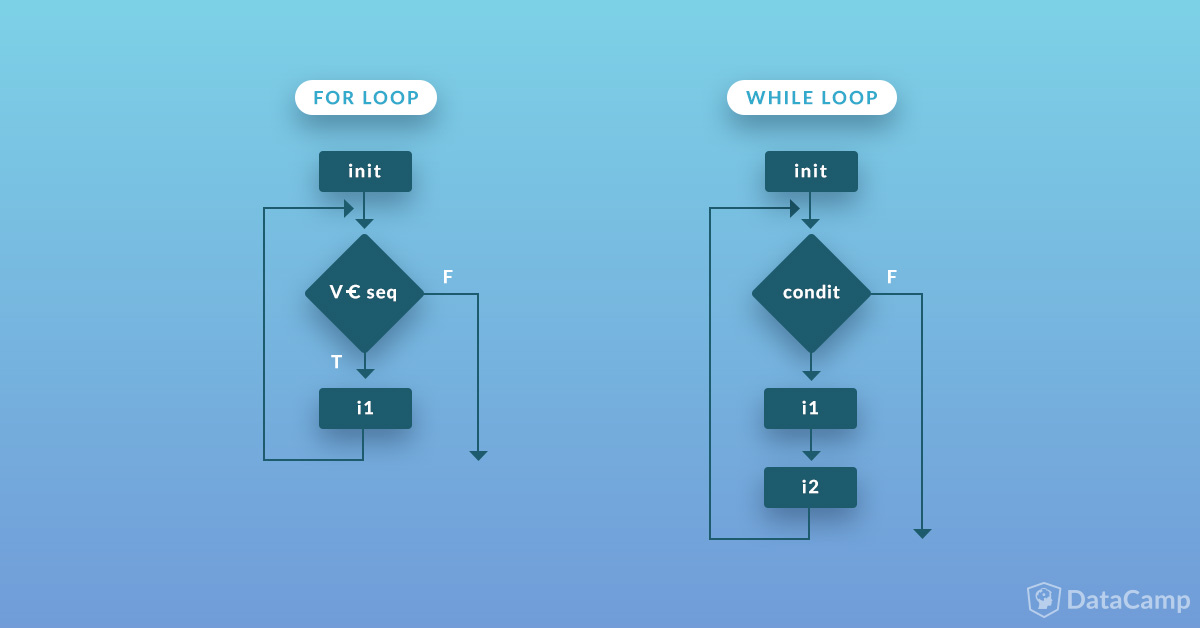
LOOPS
So far, we have learned to create programs that serves one purpose and only executes once. What if we want that program to keep executing? For instance, run multiple times in order to enter employee information for numerous employees. Perhaps we want the program to run until the user gets the answer right. Maybe we want the program to run until the user wants to quit.
Running a section of code numerous times in known as a loop. In Python, we will focus on two types of loops, the for loop and the while loop.
Range
In order to truly appreciate the for loop, we must first understand the concept of range. In Python 3.x, the syntax of the range function is:
[python]
range(x,y,z)
[/python]
where
x is your starting number
y is your ending number (non-inclusive)
z is your increment value
Let’s look at this in more detail. The code
[python]
range(5) #this will produce the values 0,1,2,3,4 (not inclusive)
range(0,5) #this will produce the values 0,1,2,3,4 (does not include the final value)
range(0,10,2) #this will produce the values 0,2,4,6,8 (again, does not include the final value)
[/python]
Sample Code
Without getting into the concept of the for loop just yet, let’s see the effect of the range function. Copy the code below and execute it. Does it create the result you expected? Change the numbers and see if you can predict what the results will be.
[python]
"""
This program will print all of the numbers stored in a range function
"""
#Using the range function with only one value. Try changing the number to see what will happen. What happens if you use negative numbers?
for x in range(7):
print(x)
#Using the range function with two values. Try changing the numbers to see what will happen. What happens if you use negative numbers?
for y in range(2,9):
print(y)
#Using the range function with three values. Try changing the numbers to see what will happen. What happens if you use negative numbers?
for z in range(3,15,3):
print(z)
[/python]
The For Loop
A for loop is used for iterating over a sequence.
This is less like the for keyword in other programming language, and works more like an iterator method as found in other object-orientated programming languages.
For loops can iterate over a sequence of numbers using the “range” and “xrange” functions. Since we are using Python 3.x, we will be using the “range” function (xrange is used in Python 2.x). The difference between range and xrange is that the range function returns a new list with numbers of that specified range, whereas xrange returns an iterator, which is more efficient. Note that the range function is zero based. More on that in a minute
The syntax for a for loop is:
[python]
for iteratingVal in sequence:
statements(s)
[/python]
Let’s look at some examples of the use of the for loop and range.
Sample Code
Copy the code below and execute it. Be sure to read the comments to see what it is that we’re trying to accomplish. Does it create the result you expected? Change the numbers and see if you can predict what the results will be.
[python]
#use a for loop to cycle through every value
#in the range
for loopValue in range(5):
print("loopValue:",loopValue)
#Create a hide-and-go seek counter for the person who is it
#They must count to 10 before they can ‘seek’
for counter in range(10):
print(counter)
print("Ready or not … here I come!")
print("Johnny says, ‘This kid can’t count good’.")
"""
Notice that in the above example that the counting began at 0? That is because range is a zero based function, meaning it starts counting from zero and not one like we are used to.
Aslo, the number 10 was not included in the range.
Finally, the line "Ready or not … here I come!" was executed every time since it is indented.
"""
#Let’s create a better version
#hsCounter 2.0 will start at one, end at ten, and only say "Ready or not" once completed
for counter in range(1,11):
print(counter)
print("Ready or not … here I come!")
#See the difference between the two?
[/python]
The For Loop – User Defined and Decreasing
In the previous examples, we allowed the programmer to create the range that will be used. What if we want to allow the user to define how long the program will run? You can use a range that uses an integer variable instead the actual integer itself. See the example below.
Sample Code
Copy the code below and execute it. Be sure to read the comments to see what it is that we’re trying to accomplish. Does it create the result you expected? Change the numbers and see if you can predict what the results will be.
[python]
#Create a program that will execute predetermined user defined countdown.
#First, get the user input
num = int(input("Please enter the countdown value: "))
#Create the for loop with the following:
#Starting at the user value
#Up to, but not including, zero
#Decrease by 1 each time
for counter in range(num, 0, -1): #note that value at the end
print (counter)
[/python]
Check Your Understanding
Before moving to the next section, let’s see how well you know the current material. Use the for loop and range function to complete the following:
- Create a program that will print every even number from 1 – 50
- Create a program that asks the user for a number and then prints out a list of all the divisors of that number.
- Create a program that will calculate the sum of all natural numbers between 1 and the user input.
- Create a program that will print the multiplication table (up to 10) of any number.
- Create a program that will produce the output “3 … 2 … 1 … Action!”
- Can you get it to print all on one line?